I recently had the chance to do a 2-hour Javascript evangelism talk at Dassault Systèmes. Unfortunately the presentation has not been recorded. I reused my the presentation I did at EPITA at the beginning and added a second part with a lot of demos. I've written down notes about the second part so you can get an idea.
Developer Tools
Web Inspector. It is integrated into Google Chrome and has all the features you would be expecting in an IDE. The console is really powerful as it lets you browse through the Javascript objects. You no longer need to write endless printing functions. You can edit the HTML and CSS without a page reload, it makes designing interfaces a lot more efficient. There is also a full panel dedicated to profiling both Javascript and DOM events.
- JSFiddle. Web programming is all about interactivity. Not only you with the program (REPL) but also with other people. Everything you do can be one link away. JSFiddle lets you try and experiment things without the need of an IDE and allows you to show it to the world easily.
- JSHint. Because Javascript, the language, has design issues and is highly dynamic, it is useful to enforce good practices and to set common programming rules when working together. Always in the spirit of the web, you can just copy and paste your code to check it. Note that JSHint can also be integrated in all major text editors and IDEs.
CSS
HTML and CSS were traditionally used to make websites and forms. We can now make completely different things.
- jmpress.js. Here's an example of how to use 3D in CSS in order to make animated presentation. One important thing to notice is how easy it is to use it. Just include jmpress.js in the page and add
data-x="-5000" data-rotate="180"
attributes to your HTML. It just works! - CSS Panic. In order to show how powerful CSS got those days, here's a game completely written in HTML + CSS. There is 0 lines of Javascript!
Canvas
Canvas is just a rectangle where you can manipulate each pixel's color.
- RayTracer. Ray Tracer is a common computer science school project. Usually, you write it on your computer and sometimes share the resulting image but you don't really share it because no one want to take the pain of compiling it on their machine. With Javascript, you can just share a link and everyone can test it!
- Canvas Rider. You can now create games in the browser. There is even a level editor implemented that follows principle of the web: interactivity. You can draw the map and move your character at the same time. When you are done, you are a single click away from saving the map and sharing it to people.
- pdf.js. The browser is now able to create applications that have always been restricted to native ones. The perfect example is this demo by Mozilla of a pdf renderer written exclusively in Javascript!
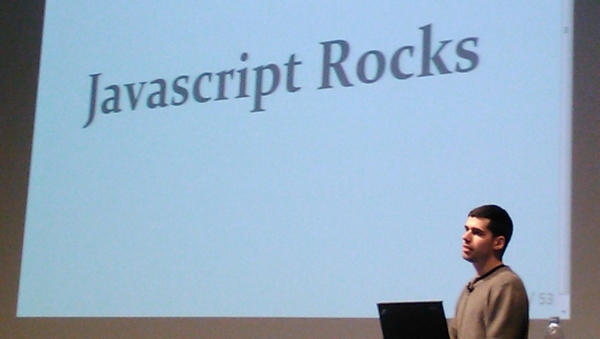
SVG
SVG let you manipulate vector graphics such as line, curve, circle ...
- Cloth Simulation. Javascript implementations have gotten fast enough to do real time constraint solving simulations such as this one. It uses SVG to easily render the graph.
- Simulated Annealing. It's another school project that gains from being written in the web. This would have probably been written in console mode, using ascii art and generating images as output. The parameters would have been entered in the command line. We can instead exploit HTML to make forms that update in real time, and SVG to render the problem and a graph to display the progress.
WebGL
WebGL is an implementation of OpenGL in the browser. It let us use the graphical card from Javascript.
- 3D Simulated Annealing. Same as previous demo but this time there is a 3D representation of the progress. It also follows the interactivity rule, you can use your mouse and WASD to explore the scene. This demo uses Web Workers to exploit multiple cores.
- WebGL Maps. The graphical card is dedicated to manipulate images, therefore you can use it to improve performance on image intensive applications such as Google Maps.
- Bevelity. Ever wondered if it was possible to write a complex application such as 3DSMax in the browser? Bevelity is an attempt to prove it true.
- Water Simulation. Another physics simulation demo with always the web plus: you can move the ball 🙂
- Hello Racer. This is one of the thousand demos that shows you a beautiful car with glossy reflects ... This one has a unique feature: you can move the car! This must not have taken more than a few dozens of lines and yet has a huge impact!
- Morph Target. Pixar also find uses for the web. Here's a demo to create facial expressions.
- Rome. Browsers now embed a video tag. Using it in combination with WebGL, Google made a wonderful 3-minute animation. You can use the mouse to interact with it. It moves the camera, pixelate the video and even make appear various monsters.
Performance
Javascript performance are impressively improving from months to months. It is now possible to write computing intensive programs and make them run at decent speed.
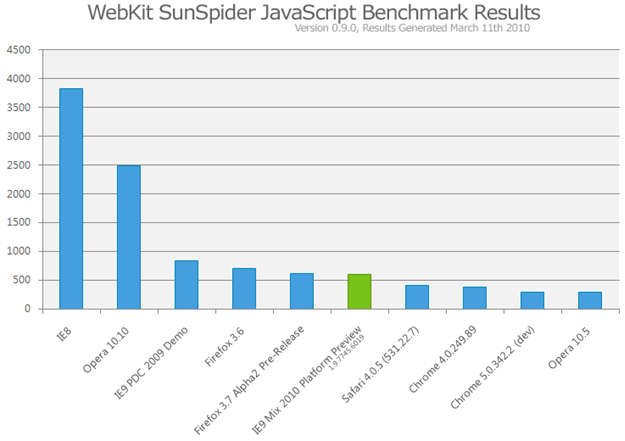
- JSPerf. If you have a doubt on which browser is faster for a specific feature or when you have two ways to do things, which one is faster, JSPerf is made for you!
- JSLinux. Typed Arrays introduced for WebGL made possible to write a CPU Virtual Machine able to run a copy of linux in under 7 seconds.
- repl.it. Emscriptem is a wonderful tool that translates LLVM assembly code into Javascript. It made possible to compile Ruby, Python, Lua and Scheme directly from their sources to Javascript.
- Broadway. Last but not least, a H.264 video decoder has been compiled to Javascript using Emscriptem. It manages to decode the sample videos at 60 frames per second. This is an exceptional feat for a scripting language!
Conclusion
It is now possible to write the same complex applications we seen in the past in the browser. And it gives one huge added value: interactivity. There's absolutely nothing to install, you just have to give a link! You can combine all the render options such as HTML, CSS, Canvas, SVG and WebGL to make your program.
The next talk I'm going to do is at the JSConf! I hope to see you there!